Mastering JavaScript: Converting Timestamps to Date
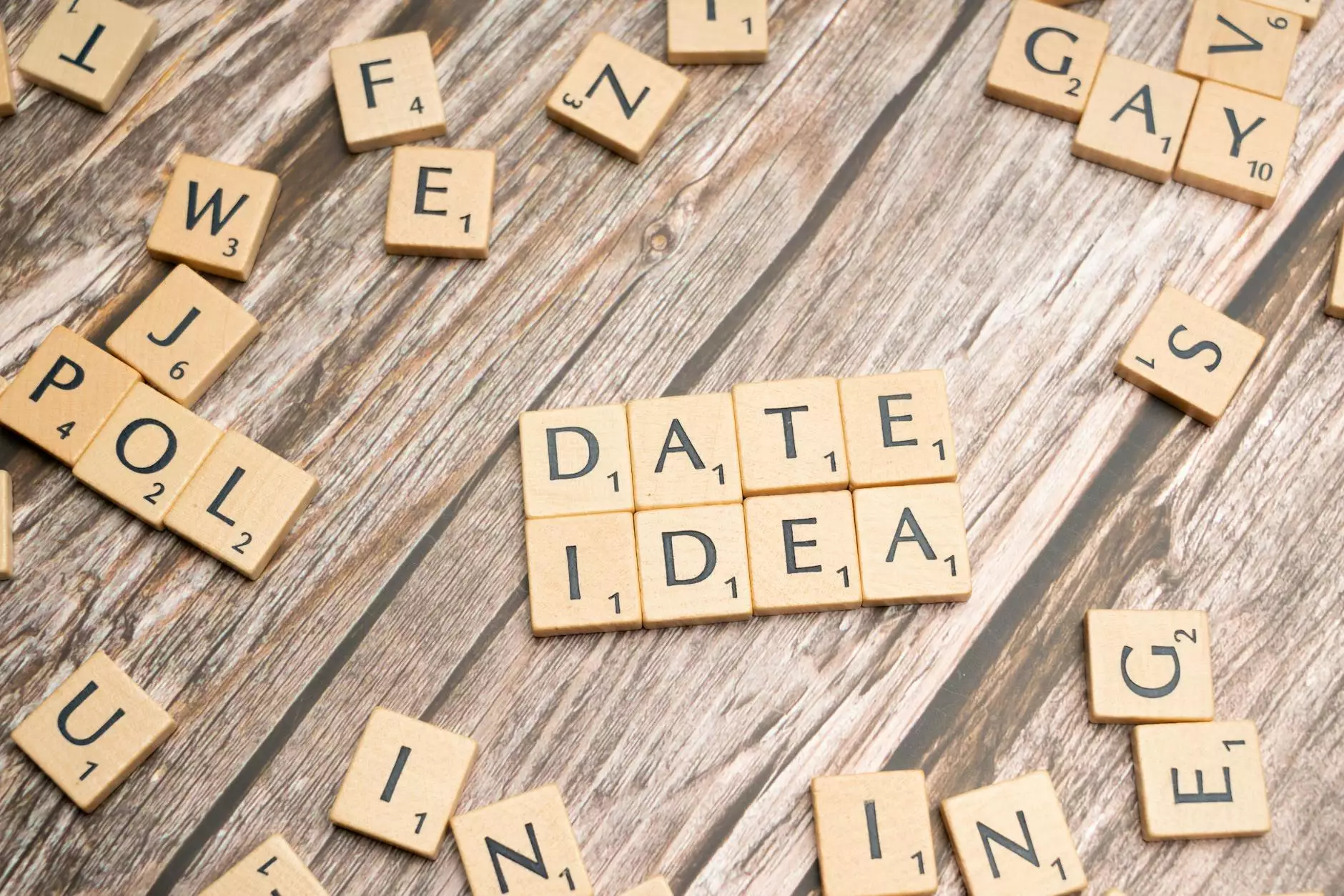
In today's fast-paced digital world, understanding how to manipulate and convert data types in JavaScript is crucial for developers. One common task that developers face is converting timestamps to human-readable dates. Whether you’re working on web design or software development, having a firm grasp of these concepts can elevate your projects to the next level.
What Is a Timestamp?
A timestamp is a numerical representation of a specific point in time. In programming, timestamps are often used to log events, record time intervals, and perform time-related calculations. In JavaScript, timestamps are commonly represented in milliseconds since the Unix Epoch, which is January 1, 1970, at UTC.
Why Convert Timestamps to Dates?
- User-Friendly Data: Converting timestamps to a date format makes it easier for users to understand when an event occurred.
- Data Manipulation: Performing operations on date objects allows for a wide range of functionalities, including comparisons and calculations.
- Internationalization: Dates can be formatted according to the user's locale for a personalized experience.
How to Convert a Timestamp to a Date in JavaScript
In order to convert a timestamp to a date in JavaScript, you can utilize the built-in Date object. Here’s a simple example:
const timestamp = 1633065600000; // Example timestamp const date = new Date(timestamp); console.log(date); // Outputs: "2021-10-01T00:00:00.000Z"Understanding the `Date` Object
The Date object in JavaScript provides numerous methods for manipulating date and time. When you create a new date instance using a timestamp, JavaScript automatically converts it to a date object:
const now = new Date(); // Current date and timeFormatting Dates for Display
While the date object is useful, it may not always display the date in an easily readable format. You may want to format the date using JavaScript's built-in methods or external libraries like date-fns or moment.js. Here are a few examples:
Using Built-in Methods
const date = new Date(timestamp); const formattedDate = date.toLocaleDateString(); // Outputs: "10/1/2021"Using External Libraries
Third-party libraries can provide powerful formatting options, making it easier to display dates:
import { format } from 'date-fns'; const formattedDate = format(new Date(timestamp), 'MM/dd/yyyy'); // Outputs: "10/01/2021"Best Practices for Working with Dates in JavaScript
When dealing with date conversions, consider the following best practices:
- Use UTC: Always perform date operations in UTC to avoid discrepancies due to time zones.
- Validate Input: Ensure that the timestamp is valid before conversion to prevent errors.
- Consistent Formatting: Define a consistent date format across your application to maintain a uniform user experience.
Handling Time Zones
Time zones can complicate date manipulation. When converting timestamps, JavaScript uses the local time zone by default. You can convert it to UTC or other time zones using methods like getUTCDate() or third-party libraries:
const utcDate = new Date(timestamp).toUTCString(); // Outputs: "Fri, 01 Oct 2021 00:00:00 GMT"Working with Date Libraries
If your application requires advanced date handling, consider using libraries designed for JavaScript date manipulation:
- Moment.js: A widely used library that provides powerful tools for parsing, validating, manipulating, and formatting dates.
- Date-fns: A lightweight alternative with a modular approach, allowing you to import only the functions you need.
Both libraries can significantly simplify working with dates and timestamps:
// Using moment.js const momentDate = moment(timestamp).format('MM/DD/YYYY'); // "10/01/2021" // Using date-fns import { format } from 'date-fns'; const formattedDate = format(new Date(timestamp), 'yyyy-MM-dd'); // "2021-10-01"Conclusion
Converting timestamps to date in JavaScript is an essential skill for developers. By using the built-in Date object along with best practices and libraries, you can handle date operations efficiently and effectively.
Remember to always keep your audience in mind. Choosing the right date format and ensuring accuracy can drastically enhance user experience. As you continue to enhance your skills in web design and software development, mastering date manipulation will undoubtedly serve you well.
For more insights and tools related to web design and software development, visit us at semalt.tools.
javascript convert timestamp to date